pgg-dev
[TypeScript] Interface, Type Alias 본문
Interface
클래스 또는 객체를 위한 타입을 지정할때 사용하는 문법
클래스를 interface 로 사용할 때
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
|
interface Shape {
getArea(): number;
// Shape interface 에는 getArea 라는 함수가 꼭 있어야 하며, 반환값은 숫자이다.
}
class Circle implements Shape {
// implements 키워드를 사용하면, 해당 클래스가 Shape interface 의 조건을 충족해야한다.
radius: number;
constructor(radius: number) {
this.radius = radius;
}
getArea() {
return this.radius * this.radius * Math.PI;
}
}
class Rectangle implements Shape {
width: number;
height: number;
constructor(width: number, height: number) {
this.width = width;
this.height = height;
}
getArea() {
return this.width * this.height;
}
}
function getRectangle(rectangle: Rectangle) {
//파라미터를 받아와야할 경우
return console.log(rectangle.getArea());
}
getRectangle(new Rectangle(3, 5));
const circle = new Circle(5);
const rectangle = new Rectangle(2, 5);
const shapes: Shape[] = [circle, rectangle];
shapes.forEach(shape => {
console.log(shape.getArea());
});
|
컴파일
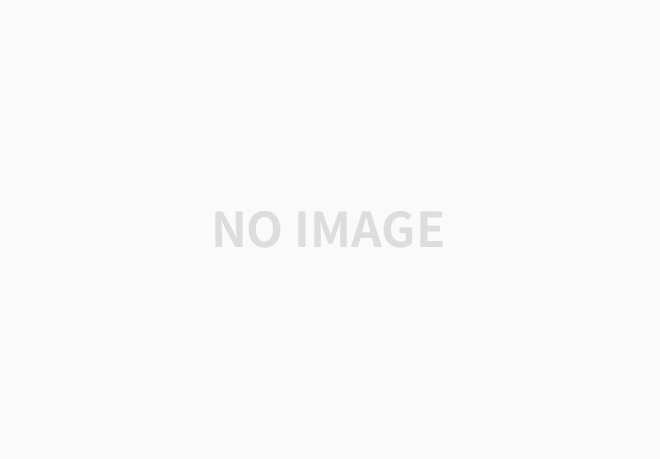
기존 코드에서
1
2
3
4
5
6
7
|
width: number;
height: number;
constructor(width: number, height: number) {
this.width = width;
this.height = height;
}
|
이런식으로 width, height 멤버 변수를 선언한 다음에 constructor 에서 해당 값들을 하나 하나 설정했다,
타입스크립트에서는 constructor 의 파라미터 쪽에 public 또는 private 를 사용하면 직접 하나하나 설정해주는 작업을 생략해줄 수 있다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
|
interface Shape {
getArea(): number;
// Shape interface 에는 getArea 라는 함수가 꼭 있어야 하며, 반환값은 숫자이다.
}
class Circle implements Shape {
// implements 키워드를 사용하면, 해당 클래스가 Shape interface 의 조건을 충족해야한다.
constructor(public radius: number) {
this.radius = radius;
}
getArea() {
}
}
class Rectangle implements Shape {
constructor(private width: number, private height: number) {
this.width = width;
this.height = height;
}
getArea() {
return this.width * this.height;
}
}
const circle = new Circle(5);
const rectangle = new Rectangle(10, 5);
console.log(circle.radius);
console.log(rectangle.width);
const shapes: Shape[] = [new Circle(5), new Rectangle(10, 5)];
shapes.forEach(shape => {
});
|
public 으로 선언된 값은 클래스 외부에서 조회 할 수 있으며 private으로 선언된 값은 클래스 내부에서만 조회 할 수 있다.
따라서 위 코드에서는 circle 의 radius 값은 클래스 외부에서 조회 할 수 있지만, 33행의 rectangle 의 width 또는 height 값은 클래스 외부에서 조회 할 수 없다.
일반 객체를 interface 로 사용할 때
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
interface Person {
name: string;
age?: number;
// 물음표는, 설정을 해도 되고 안해도 되는 값을 표시한다.
}
interface Developer {
name: string;
age?: number;
skills: string[];
}
const person: Person = {
name: "김사람",
age: 20
};
const expert: Developer = {
name: "김개발",
skills: ["javascript", "react"]
};
|
Person 과 Developer 가 형태가 유사하다.
이럴 땐 interface 를 선언 할 때 다른 interface 를 extends 키워드를 사용해서 상속받을 수 있다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
interface Person {
name: string;
age?: number;
// 물음표는, 설정을 해도 되고 안해도 되는 값을 표시한다.
}
interface Developer extends Person {
skills: string[];
}
const person: Person = {
name: "김사람",
age: 20
};
const expert: Developer = {
name: "김개발",
skills: ["javascript", "react"]
};
const people: Person[] = [person, expert];
|
Type Alias
type 은 특정 타입에 별칭을 붙이는 용도로 사용한다.
이를 사용하여 객체를 위한 타입을 설정할 수도 있고, 배열, 또는 그 어떤 타입이던 별칭을 지어줄 수 있다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
type Person = {
name: string;
age?: number;
// 물음표는, 설정을 해도 되고 안해도 되는 값을 표시한다.
};
// & 는 Intersection 으로서 두개 이상의 타입들을 합쳐준다.
type Developer = Person & {
skills: string[];
};
const person: Person = {
name: "김사람"
};
const expert: Developer = {
name: "김개발",
skills: ["javascript", "react"]
};
type People = Person[];
// Person[] 를 이제 앞으로 People 이라는 타입으로 사용 할 수 있다.
const people: People = [person, expert];
type Color = "red" | "orange" | "yellow";
const color: Color = "red";
const colors: Color[] = ["red", "orange"];
|
type 과 interface
클래스와 관련된 타입의 경우엔 interface 를 사용하는게 좋고, 일반 객체의 타입의 경우엔 그냥 type을 사용해도 무방하다 사실 객체를 위한 타입을 정의할때 무엇이든 써도 상관 없는데 일관성 있게 써야한다.
[참고]
https://medium.com/@martin_hotell/interface-vs-type-alias-in-typescript-2-7-2a8f1777af4c
https://joonsungum.github.io/post/2019-02-25-typescript-interface-and-type-alias/
'TypeScript' 카테고리의 다른 글
[TypeScript] 타입스크립트에서 React Functional Component 사용하기 (0) | 2021.01.18 |
---|